使用dynamic簡化Json.NET JObject操作
| | 5 | | 57,393 |
不知是RSS ATOM錯亂還是怎麼的,feedly RSS閱讀器冒出一篇Rick Strahl 2012的老文章 Using JSON.NET for dynamic JSON parsing ,讓我大吃一驚,發現自己一直用JProperty的笨拙方法處理動態JSON物件,其實結合dynamic就能大幅簡化。莫名其妙讀到兩年多前的文章,是老天爺的安排吧?擔心不順從天意會遭天譴,特筆記分享之。XD
以下範例示範使用強型別物件、JObject+JProperty、JObject+dymanic三種不同做法,處理JSON反序列化及序列化。
排版顯示純文字
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace JsonNetLab
{
class Program
{
static void Main(string[] args)
{
lab1();
lab2();
lab3();
lab4();
lab5();
lab6();
Console.Read();
}
static string jsonSrc =
@"{
""d"": ""2015-01-01T00:00:00Z"",
""n"": 32767,
""s"": ""darkthread"",
""a"": [ 1,2,3,4,5 ]
}";
public class foo
{
public DateTime d { get; set; }
public int n { get; set; }
public string s { get; set; }
public int[] a { get; set; }
}
//反序列化 方法1 定義強型別物件接收資料
static void lab1()
{
foo f = JsonConvert.DeserializeObject<foo>(jsonSrc);
Console.WriteLine("d:{0},n:{1},s:{2},a:{3}", f.d, f.n, f.s,
string.Join("/", f.a));
}
//反序列化 方法2 使用JObject、JProperty、JArray、JToken物件
static void lab2()
{
JObject jo = JObject.Parse(jsonSrc);
DateTime d = jo.Property("d").Value.Value<DateTime>();
int n = jo["n"].Value<int>();
string s = jo["s"].Value<string>();
int[] ary = jo["a"].Value<JArray>()
.Select(o => o.Value<int>()).ToArray();
Console.WriteLine("d:{0},n:{1},s:{2},a:{3}", d, n, s,
string.Join("/", ary));
}
//反序列化 方法3 使用dynamic
static void lab3()
{
JObject jo = JObject.Parse(jsonSrc);
dynamic dyna = jo as dynamic;
var ary = ((JArray)jo["a"]).Cast<dynamic>().ToArray();
Console.WriteLine("d:{0},n:{1},s:{2},a:{3}", dyna.d, dyna.n, dyna.s,
string.Join("/", ary));
}
//序列化 方法1 使用強型別
static void lab4()
{
foo f = new foo()
{
d = new DateTime(2015, 1, 1),
n = 32767,
s = "darkthread",
a = new int[] { 1, 2, 3, 4, 5 }
};
Console.WriteLine("JSON:{0}", JsonConvert.SerializeObject(f));
}
//序列化 方法2 使用JObject、JPorperty、JArray
static void lab5()
{
JObject jo = new JObject();
jo.Add(new JProperty("d", new DateTime(2015, 1, 1)));
jo.Add(new JProperty("n", 32767));
jo.Add(new JProperty("s", "darkthread"));
jo.Add(new JProperty("a", new JArray(1, 2, 3, 4, 5)));
Console.WriteLine("JSON:{0}", jo.ToString(Formatting.None));
}
//序列化 方法3 使用dynamic
static void lab6()
{
dynamic dyna = new JObject();
dyna.d = new DateTime(2015, 1, 1);
dyna.n = 32767;
dyna.s = "darkthread";
dyna.a = new JArray(1, 2, 3, 4, 5);
Console.WriteLine("JSON:{0}", dyna.ToString(Formatting.None));
}
}
}
相較之下,使用dynamic動態型別,效果與使用JProperty存取相同,但程式碼簡潔許多,未來在.NET 4+環境就決定用它囉~
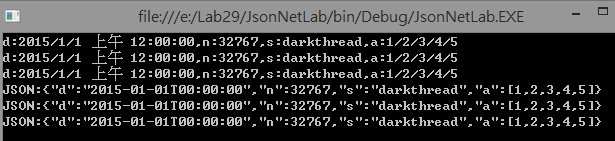
Comments
# by Fish
方法1和2標記似乎重覆了?
# by Jeffrey
to Fish, 嘿,猶豫不決要編方法1-6還是序列化跟反序列化各編方法1-3,結果一團亂。謝謝提醒,已重新整理。
# by wellxion
好奇問問... 效能差距如何呢:D?
# by edr
有用
# by River
thx for sharing